In a Computation item, a mathematical computation is executed on the phone of the client, based on the client’s previous answers. This computation item or computation is not a real question as the script will just run on the phone of the participant/client without him/her noticing. The result of the computation item has the same functionalities as any other answer from a participant.
These computations happen in real-time. No connection to the internet is necessary. Computation items give the possibility to make adaptive questionnaires.
Specifically, computation items can be used to:
- Compute a sum score or average score of a questionnaire.
- Use advanced conditional logic.
- Compute new constructs that result from the client’s previous answers.
- Randomize questions.
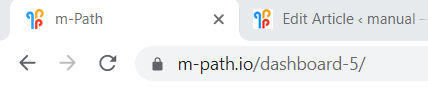
Pseudo R
The scripting language used to write computations is based on R. Therefore it’s called Pseudo R. R was chosen as this is probably the language most psychologists or even statisticians are familiar with. The goal is to implement as many functionalities from R to make real-time computations in m-Path as useful as possible.
Example questionnaire ‘computation examples’
Most computations in this article can be tested by using the ‘computation examples R‘ questionnaire from Freud which can be found in the m-Path questionnaire library. This questionnaire starts with two questions:
- How happy are you now? (label ‘happy’)
- How sad are you now? (label ‘sad’)
In the last question, all answers from all computation questions are shown using piped text.
How to create a computation item?
- Choose the Computation question type.
- Choose the label of the computation, for example ‘happyPlus50’.
- Make sure to set ‘code script‘ in pseudo R. If you are not able to set the script to pseudo R, you are still working with an older dashboard. The old computation item is explained here.
- Type the Mathematical expression, for example happy+50. The value of ‘happyPlus50’ will then be set to 50 plus the answer of the question with label ‘happy’.
- [optional] Fill in the minimum and maximum value for visualization. If this computation question needs to be visualized in a plot, m-Path needs to know its minimum and maximum values. As the minimum and maximum value of the ‘How happy are you now?’ question are 0 and 100, the minimum and maximum value of happyPlus50 are 50 and 150.
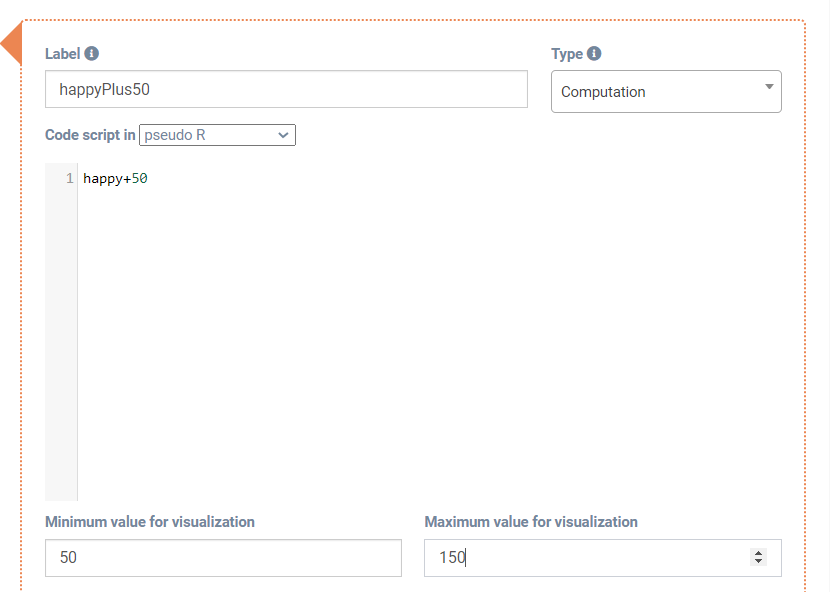
Possible mathematical expressions
Normal mathematical expressions
A normal mathematical expression is an expression such as 5+3*2 +4/2. This will give 13 as a result (see question with label normalMathExpression_1 in example). An absolute value is also possible. For example (-5)^2 will result in 25 (see questions with label ‘normalMathExpression_2’).
Use answers from previous questions
Answers from previous questions (from the same questionnaire) can also be used in mathematical expressions. To access these answers, the label of the previous questions can be used in the expression. For example happy-sad can be used to subtract ‘sad’ from ‘happy’ to get some general affect (see label question with label ‘happyMinusSad’ in example).
Labels from previous computation questions can also be used (E.g. happy+happyPlus50 in question with label ‘happyPlus50Re-use’).
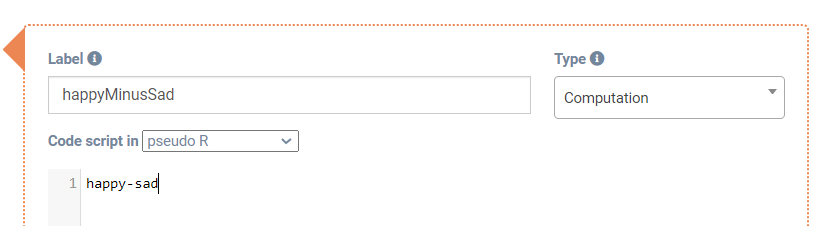
Scripts or multiline mathematical expressions
Like in R, it is also possible to create expressions that span over multiple lines (so-called scripts). In each line a new variable can be declared. Declaring a variable can be done using the = or the <- sign. In the following script (found in question with label ‘scriptExample’) three new variables will be set:
scriptComputation1<-100
scriptComputation2=happy*4
scriptComputation3=scriptComputation2-100
This script will result in 3 answers (with labels ‘scriptComputation1’, ‘scriptComputation2’ and ‘scriptComputation3’). All of them can be used in later questions.
Note that ‘scriptExample’ is the label of the question, but the variable itself is not set. There will only be an answer with label ‘scriptExample’ if
- There is an ans expression. This is an expression where NO new variable is explicitly set, like 5*happy (but not newVariable = 5*happy). The answer of the overall label of the question will be set to the last ans expression.
- A variable is set with label ‘scriptExample’. For example sctriptExample=70.
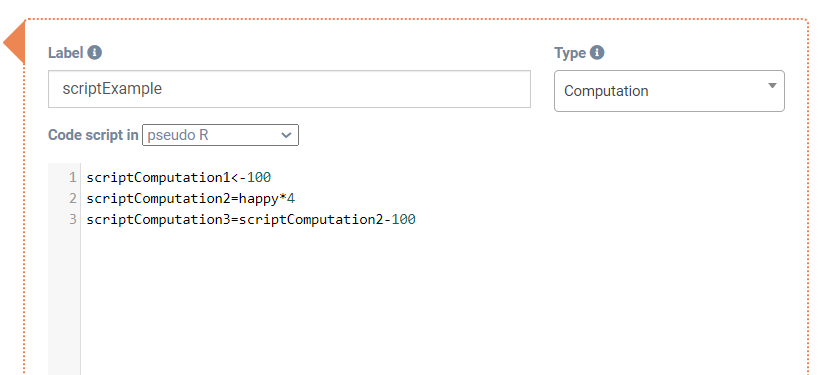
Comments in script
It is also possible to add comments in the script. Everything after # will be ignored.
# this statement will be ignored in the script
Vectors or arrays
It is possible to create an array using the c() command. The following script will create a new array and subsequently add a number to it:
arrayExample=c(1,2,3,4)
arrayExample=c(arrayExample,5)
Strings
You can define strings using single or double quotations marks.
text1 = 'Text1'
text2 = "Text2"
Using functions
Many functions from R can already be used in m-Path. Only the base functions can however be used, additional settings can not be included. For example mean(c(1,2,3)) is possible but mean(c(1,2,3),trim=0.1) is not.
Additionally, also some functions that are not in R are included. For example, the reverse function reverses the score, based on the scale of the variable. This can be very useful when creating a construct that includes reverse scores.
All functions are shown in the example questionnaire in the question with label ‘allFunctions’.
mean(c(happy,sad))
: the mean of ‘happy’ and ‘sad’.median(c(happy,sad))
: the median of ‘happy’ and ‘sad’.nanmean(c(happy,sad,other))
: the mean of ‘happy’, ‘sad’ and ‘other’. Exclude variables that do not exist (which is the case for ‘other’ in the example). At least a single variable has to exist for this function to work.max(c(happy,sad))
: the maximum of ‘happy’ and ‘sad’.min(c(happy,sad))
: the the minimum of ‘happy’ and ‘sad’.sum(c(happy,sad))
: for the sum of ‘happy’ and ‘sad’.sqrt(happy)
: the square root of ‘happy’log(happy)
: the log of ‘happy’abs(happy)
: the absolute value of ‘happy’ceil(happy)
: the value of ‘happy’ ceiledfloor(happy)
: the value of ‘happy’ floorround(happy)
: the value of ‘happy’ roundedquantile(c(happy,sad),0.5)
: the 50% percentile of the array.length(c(happy,sad))
: the length of the arrayrnorm(3)
: creates an array of 3 random variables (normally distributed)runif(3)
: creates an array of 3 random variables (uniformly distributed)is.na(happy)
: returns 1 if happy is not available and 0 if it existsreverse(happy)
: reverses the score of happy. In this case, as happy is on a scale from 0 to 100 it will result in 100-happy. If it would have been a seven point likert scale, it would have resulted in 7-happy+1 (such that the reverse score goes from 7 to 1).as.character(happy)
: converts a number to a string.nchar('test')
: counts the characters in a string. This example will return 4.paste('test','1')
: this will paste two strings together. This example will result in ‘test1’.substr('test5',2,3)
: this will generate a substring. This example will result in ‘es’.tolower('Test')
: transforms a string to lower case. In this case the result would be ‘test’.grepl('pattern','string that contains pattern')
: checks if a string contains a pattern. In this case the output would be 1 (true).as.Date(time)
: this will format a time (seconds since epoch) into a date format. It outputs a string in format ‘year-month-day’. It will also transform UTC time to current time. Use as.Date(Sys.time) for the current date.as.weekday(time)
: this will return what day of the week this timestamp indicates in an integer value (with Monday being 1 and Sunday being 7).as.day(time)
: this will return what day of the month this timestamp indicates in an integer value.as.hours(time)
: this will format a time (seconds since epoch) into the hours of a day. It will also transform UTC time to current time. Use as.hours(Sys.time) for the current hours of the day. Also see example 8.as.minutes(time)
: this will format a time (seconds since epoch) into the minutes of a day. It will also transform UTC time to current time. Use as.minutes(Sys.time) for the current hours of the day. Also see example 8.as.seconds(time)
: this will format a time (seconds since epoch) into the hours of a day. It will also transform UTC time to current time. Use as.seconds(Sys.time) for the current seconds of the day. Also see example 8.is.skipped(happy)
: returns 1 (true) if question with label happy was skipped. This is useful for questions that can be skipped. See ’empty answer test’ questionnaire for examples.is.timedout(happy)
: returns 1 (true) if question with label happy was timed out. This is useful for questions with a deadline. See ’empty answer test’ questionnaire for examples.is.empty(happy)
: returns 1 (true) if question with label happy was empty. If a question was not yet filled in and did not have a valid starting value when it was skipped or timed out, the answer is empty. See ’empty answer test’ questionnaire for examples.match(a, b)
: returns a vector of the positions of (first) matches of its first argument in its second. For example match(3,c(1,4,5,3,6)) will return 4.as.responsetime(happy)
: gives the response times of the question with label happy. Using this feature, it is possible to in real time adapt a questionnaire to a participant that is answering to quickly.as.question(happy
): gives you the question related to the question with label happy. For example ‘how happy are you now?’.sample.int(5,1)
: samples from an integers. In this case, one integer is generated from the numbers 1 to 5. In case of sample.int(5,2), two integers are generated (without replacement).shewhart.UCL(a)
: if a is a list or vector of numbers, the shewhart.UCL(a) gives you the upper bound for Shewhart procedures of process control.shewhart.LCL(a)
: if a is a list or vector of numbers, the shewhart.LCL(a) gives you the lowerbound for Shewhart procedures of process control.seq(1,5)
: gives you a sequence of integers 1 to 5.var(a)
: if a is a list or vector of numbers, var gives you the variance of these numberssd(a)
: if a is a list or vector of numbers, sd gives you the standard deviation of these numbersdistm(a,b)
: if a is a vector with a latitude and a longitude and b is a vector with a latidude and a longitude, distm will give you the distance between both points using the Haversine formula in meters. For example distm(c(51.04, 4.488),c(52.14, 3.523)). This can be useful if a location sensor is used.which(a)
: returns the position or the index of the value for which a is equal to 1. For example which(c(1,0,1,0)) would return c(1,3). As such which(happyList>50) returns a list of indexes where the numbers in happyList are larger than 50.
Combination of the above
Use the () brackets to work with nested expressions. For example statement mean(max(happy,70),sad )*2 can be found in the example questionnaire under the question with label ‘bracketExample’.
Logical expressions
The following signs can be used to create a logical expression or an equation: >,<,==,<= and >=. If the expression is true, the result will be 1, if not, the result will be 0. Additionally, logical operators like && and || can be used.
For example happy>sad && sad > 50 (see question with label ‘logicalStatement’ in example questionnaire) will result in 1 only if the answer of ‘happy’ is bigger than the answer of ‘sad’ and ‘sad’ is higher than 50.
If else statements
It is also possible to use if else statements in the script. The following will set ‘ifElseVariable’ to 1 if happy is bigger than sad. Otherwise it will be set to 0 (see question with label ‘ifElseVariable’).
if(happy>sad){
ifElseVariable=1
}else{
ifElseVariable=0
}
Constants or predefined variables
It is possible to call predefined variables:
Sys.time
: the current time in seconds. This is in UTC time. If the current hour in local time is needed, one can use as.hours(Sys.time) (see functions above).system_nToday
: The number of notifications answered on that day by the user, including the current questionnaire. If this is equal to one, it means that the user answering the first notification of the day. If the same notification is answered twice, this number will not increase. This includes all scheduled notifications and follow-up notifications (but not manually sent notifications).system_nTodayL
: The number of questionnaires filled in on that day by the user, including the current questionnaire. If the same notification is answered twice, this number will increase.system_nThisWeek
: The number of notifications answered in the previous week. If the same notification is answered twice, this number will not increase. This includes all scheduled notifications and follow-up notifications (but not manually sent notifications).system_progToday
: the percentage of notifications answered by the participant on that day. Equals to system_nToday divided by the total number of notifications that are expected to be sent on that day.system_maxstreak
: The amount of consecutive days the participant has filled in their questionnaires.
Conditional on computation question
Conditions can be defined for computation questions. The interactions or questions in the yes condition will only be shown if the result of the computation question was equal to 1. More specifically, it will check the answer from the label of the computation question. In the following example, this label is conditionalComputation.
If conditionalComputation is equal to 1, the yes condition will be triggerd. If conditionalComputation is 0, the no condition will be triggerd.
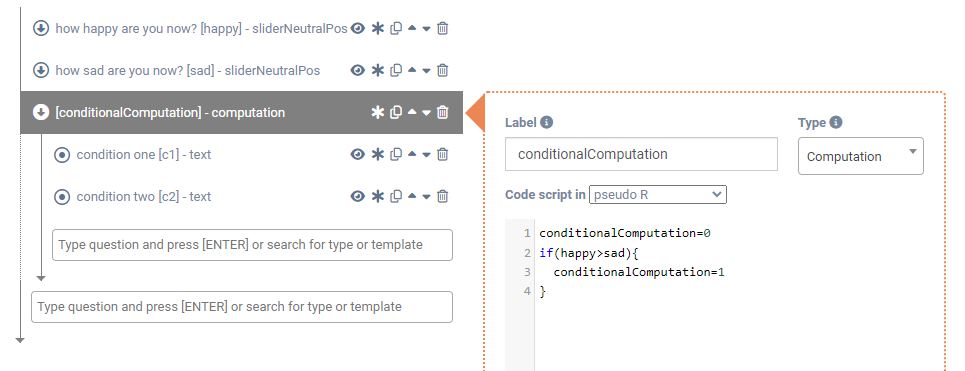
E.g. If the expression of the computation question was
conditionalComputation=0
if(happy>sad){
conditionalComputation=1
}
the condition will be true if happy was actually bigger than sad, which will result in the answer 1 for the computation question (with label conditionalComputation). This is shown in the question with label ‘conditionalComputation‘ in the example questionnaire.
Logical statements can be used to add complex logic to the conditional structure of the questionnaire.
Answer validation
Using a command statement conditional on a computation question makes it possible to validate specific answers. For example:
- Go back to the previous question when no answer option was given
- Go back to the previous question when the answer is not long enough (in case of a text based answer).
Some examples of this are given in the Answer validation example questionnaire in the library.
Use answers from previous questionnaires (load and save answers)
Using load and save statements, answers from previous questionnaires (at previous time points) can be loaded to execute more advanced expressions and (e.g. compute an average over time or even use time series models). This can be done by loading an answer beforehand using a load answer question type. Afterwards the answer can be saved again using the save answer question type. Regardless of where the save command is located in the script or questionnaire, the save will happen at the very end of the questionnaire, using the last available value of the answer/variable. This means that any change made to it later in that questionnaire will be saved as well.
It is also possible to incorporate load and save statements in a script. The following script (shown in saveLoadExample in the example questionnaire), a variable or answer ‘previousHappy’ is loaded. If this answer is not yet available it is set to 50. Subsequently, ‘delta’ is set to the difference between (current) ‘happy’ and ‘previousHappy’. Finally, ‘previousHappy’ is overwritten and saved again.
load("previousHappy")
if(is.na(previousHappy)){
previousHappy=50
}
delta=happy-previousHappy
previousHappy=happy
save("previousHappy")
For testing purposes (see bellow) it is also possible to unsave variables.
unsave("previousHappy")#unsaves or clears the variable previousHappy
unsave("") #unsaves or clears all saved variables
Testing
Using computation questions it is possible to add very complex logic to interactions and questionnaires. However, due to this complexity it may be difficult to debug. Following tips may improve the ease of testing:
- Enable debug mode. In debug mode, it is possible to look at all outcomes, also from computation items, during a questionnaire. Therefore, it is possible to immediately see the results of a computation, without having to download the data from the dashboard.
- Using the unsave(“”) command all saved variables are cleared. Now you don’t need to recreate a new participant to simulate going through a questionnaire for the first time, when no variables can be loaded yet.
Examples
Example 1: Intervention after low depression construct
In this example (see ‘constructExample’ in the example questionnaire) a construct is computed. Conditional on this construct an intervention is proposed.
averageAffect=mean(happy,reverse(sad))
if(averageAffect<20){
constructExample=1
}else{
constructExample=0
}
Additionally, the questionnaire ‘Affect construct computation example’, which can be found in the library, also applies this logic. First, multiple questions are asked about affect. Then a construct is computed based on these affect scores.
affect_construct=mean(c(affect_1,affect_2,affect_3,reverse(affect_4),reverse(affect_5)))
Finally, a different text is shown based the construct.
Example 2: Ask a question in 20% of the questionnaires
In this script (see ‘randomExample’ in example questionnaire) a conditional question is asked in 20% of the cases. This can be done using following expression: runif(1)<0.2.
Example 3: Propose intervention when participant scores relatively low
In this example (see question with label ‘relativelyLow’) an intervention is done when the current happy score is relatively low (within person). The script is explained in the comments (parts after #)
load("allHappyScores") # load all previous happy scores
if(mean(is.na(allHappyScores))){
allHappyScores=c() # set allHappyScores to empty array if it doesn't exist
}
allHappyScores=c(allHappyScores,happy) # add current happy score to array
lowScoreCut=quantile(allHappyScores,0.1) # compute 10% percentile
#only do when there are at least 10 measurements and the current score is low
if(happy<=lowScoreCut && length(allHappyScores)>=10){
relativelyLow=1
}else{
relativelyLow=0
}
save("allHappyScores") # save array again
Example 4: Compute running average
In this script (see ‘runningAverage’ in example questionnaire), a running average is computed. This script has no need for arrays, which is therefore less heavy on the memory needs computation requirements.
load("N") # load N, the total amount of measurments
load("sumHappy") # load sumHappy, the sum of all previous happy answers
if(is.na(N)){
N=0 # set N to 0 when it doesnt exist yet
}
if(is.na(sumHappy)){
sumHappy=0 # set sumHappy to 0 when it doesnt exist yet
}
N=N+1 # increase N
sumHappy=sumHappy+happy # add happy to sum
runningAverage=sumHappy/N # compute average
# save variables
save("N")
save("sumHappy")
Example 5: A random number test
This example can be used in a cognitive test where the app user has to remember a random number. First a random number is generated. Second, this number is visualized in the app. Third, the user can input a number. Lastly a computation item compares the inputted number to the original number to test if it was correct. The whole example can be found in the ‘Random Number Test’ questionnaire in the library. To set a timer on each question see the ‘has deadline‘ setting.
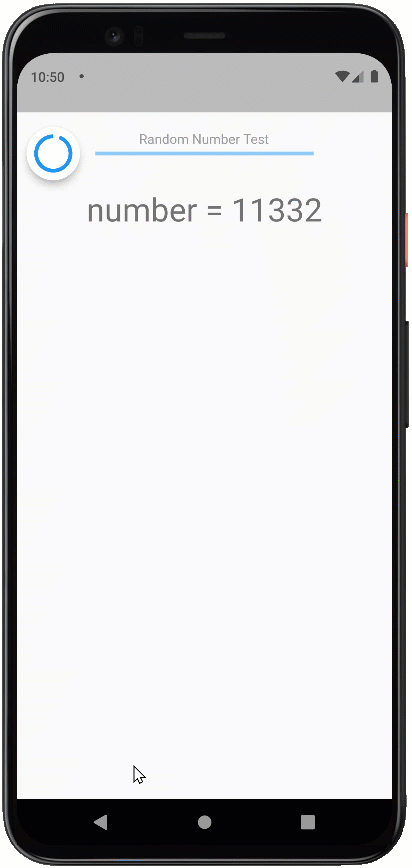
In this example we will first create a random number with a specific length.
lengthNumber=5 # the length of the number
# generate anumber with this length
range=(10^lengthNumber-1)-(10^(lengthNumber-1))
randomNumber=(10^(lengthNumber-1))+round(runif(1)*range)
Subsequently this random number will be shown using piped text. The question statement that was used is the following: number = @INSERT{[randomNumber]}.
The app user can then fill in an open question with label ‘guessNumber’.
In the last step the inputted number needs to be compared to the original number. As the inputted number is a string we also need to convert the random number to a string to compare both.
guessNumber==as.character(randomNumber)
Conditional on this the user will see if he remembered the number correctly or not.
Example 6: Time test
In this it is tested if a certain amount of time has already passed since the last notification. The example can be found in the questionnaire in the library named ‘time check computation’. The main computation code is found in the item with label ‘tooLong’:
load('previousTime')
if(is.na(previousTime)){
previousTime=0
}
currentTime=Sys.time
timeWindow=currentTime-previousTime
if(timeWindow>10){
tooLong=1
}else{
tooLong=0
}
First, ‘previousTime’ is loaded. If this variable doesn’t exist it is set to 0. Than the current time is extracted from the phone (in seconds). If the elapsed time (timeWindow) is larger than 10, tooLong is set to 1. In this case, the conditional logic of the questionnaire will see tooLong as true and the participant will see the text ‘more than 10 seconds elapsed since previous beep’ when the example questionnaire is used. At the end of the questionnaire, in a new computation item, previousTime is set to currentTime and saved. This way, the next time ‘previousTime’ is loaded in a questionnaire, it is correct.
previousTime=currentTime
save('previousTime')
Example 7: First beep of the day test
In this easy example it is tested if a beep is the first notification of a day. This can be used such that questions about the previous night are only asked once, and only asked on the first notification that is filled in of the day. One just has to create a computation question with following script.
system_nToday==1
As explained above, system_nToday counts the number of notifications answered on a specific day.
Conditional on this computation question, the additional questions can be posed. For example, when a participant misses the first four questionnaires/notifications of a day but answers the fifth, the additional questions will be asked on the fifth.
Example 8, add a question if time after 19:30
In this example, an additional question is added if a survey is answered after 19:30. First we get the current time. Then we check if this time is after 19:30 (local time). We set doAdditionalQuestion to 1 if that is the case. Make sure to also set doAdditionalQuestion as the label of the question if you want to pose conditional questions on this. This example can be found in the libary under time check example.
currentTime=Sys.time
doAdditionalQuestion=0
if(as.hours(currentTime)>=20){
doAdditionalQuestion=1
}
if(as.hours(currentTime)>=19 && as.minutes(currentTime)>=30){
doAdditionalQuestion=1
}
Often, it is unnecessary to use a computation to check the time of the participant. It is possible to create a schedule that includes multiple questions. Therefore, it is perfectly possible to create an evening questionnaire (send after 19:30) and other questionnaires (send before 19:30).
Additionally, the current computation will also set doAdditionalQuestion to 0 if the survey is answered after 24:00.
Example 9, ask additional question conditional on loaded variable
It is possible to first ask questions in a previous questionnaire and than later ask questions conditional on this answer. For example, in the intake questionnaire you can have a yes/no question ‘Do you have a partner?‘ with label partner. This answer can later be saved in the intake questionnaire using the save answer type or the following computation statement:
save('partner')
In a later questionnaire, it is possible to ask questions conditional on this answer using following code in a computation question with label doExtraQuestion.
load('partner') # load the answer
# give the answer a value if it cannot be loaded
if(is.na(partner)){
partner=0
}
doExtraQuestion=(partner==1)
An example of this setup is shown in library questionnaires save load example part 1 (for the saving of the answer) and save load example part 2 (for the loading of the answer and the conditional question).